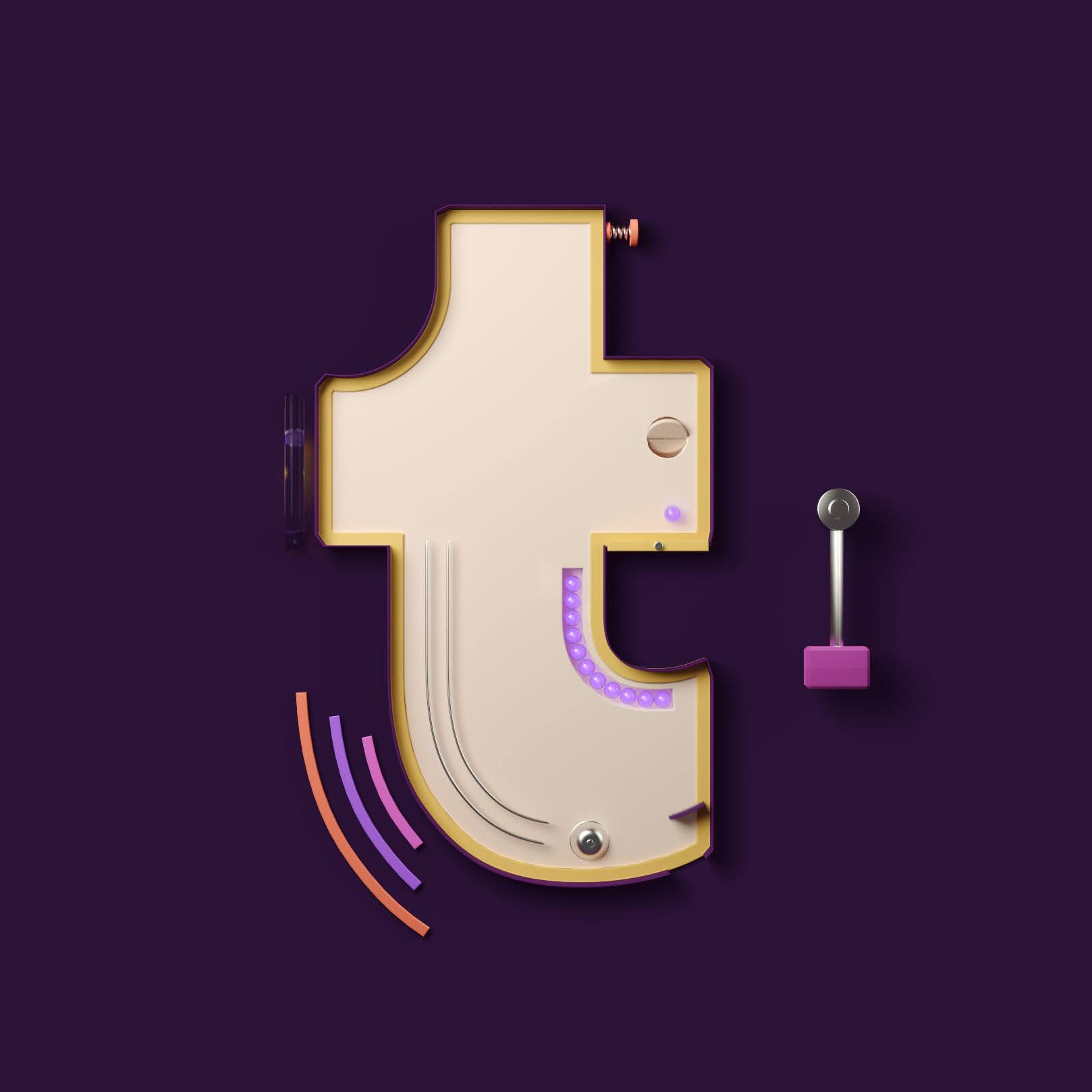
Your Experience
Implement
Use Toggl to implement your best workflow according to your needs.
Extend
Extend on our functionalities and customize using our easy and intuitive REST API.
Quick Start
Authenticate
- cURL
- Go
- Ruby
- JavaScript
- Python
- Rust
curl -X POST https://api.track.toggl.com/api/v9/me/sessions \
-H "Content-Type: application/json"
req, err := http.NewRequest(http.MethodPost,
"https://api.track.toggl.com/api/v9/me/sessions")
if err != nil {
print(err)
}
req.Header.Set("Content-Type", "application/json; charset=utf-8")
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
print(err)
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
print(err)
}
fmt.Print(string(body))
require 'net/http'
require 'uri'
require 'json'
uri = URI('https://api.track.toggl.com/api/v9/me/sessions')
http = Net::HTTP.new(uri.host, uri.port)
req = Net::HTTP::Post.new(uri.path)
req['Content-Type'] = "application/json"
res = http.request(req)
puts JSON.parse(res.body)
fetch("https://api.track.toggl.com/api/v9/me/sessions", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
})
.then((resp) => resp.json())
.then((json) => {
console.log(json);
})
.catch(err => console.error(err));
import requests
from base64 import b64encode
data = requests.post('https://api.track.toggl.com/api/v9/me/sessions', headers={'content-type': 'application/json'})
print(data.json())
extern crate tokio;
extern crate serde_json;
use reqwest::{Client};
use reqwest::header::{CONTENT_TYPE};
#[tokio::main]
async fn main() -> Result<(), reqwest::Error> {
let client = Client::new();
let json = client.request(Method::POST, "https://api.track.toggl.com/api/v9/me/sessions".to_string())
.header(CONTENT_TYPE, "application/json")
.send()
.await?
.json()
.await?;
println!("{:#?}", json);
Ok(())
}
Start Tracking
- cURL
- Go
- Ruby
- JavaScript
- Python
- Rust
curl -X POST https://api.track.toggl.com/api/v9/time_entries \
-H "Content-Type: application/json" \
-d '{"time_entry":{"description":"Hello Toggl","tags":["billed"],"duration":1200,"start":"1984-01-03T19:15:05.000Z","pid":123}}'
bytes, err := json.Marshal('{"time_entry":{"description":"Hello Toggl","tags":["billed"],"duration":1200,"start":"1984-01-03T19:15:05.000Z","pid":123}}')
if err != nil {
print(err)
}
req, err := http.NewRequest(http.MethodPost,
"https://api.track.toggl.com/api/v9/time_entries", bytes.NewBuffer(bytes))
if err != nil {
print(err)
}
req.Header.Set("Content-Type", "application/json; charset=utf-8")
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
print(err)
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
print(err)
}
fmt.Print(string(body))
require 'net/http'
require 'uri'
require 'json'
uri = URI('https://api.track.toggl.com/api/v9/time_entries')
http = Net::HTTP.new(uri.host, uri.port)
req = Net::HTTP::Post.new(uri.path)
req['Content-Type'] = "application/json"
req.body = {"time_entry":{"description":"Hello Toggl","tags":["billed"],"duration":1200,"start":"1984-01-03T19:15:05.000Z","pid":123}}.to_json
res = http.request(req)
puts JSON.parse(res.body)
fetch("https://api.track.toggl.com/api/v9/time_entries", {
method: "POST",
body: {"time_entry":{"description":"Hello Toggl","tags":["billed"],"duration":1200,"start":"1984-01-03T19:15:05.000Z","pid":123}},
headers: {
"Content-Type": "application/json"
},
})
.then((resp) => resp.json())
.then((json) => {
console.log(json);
})
.catch(err => console.error(err));
import requests
from base64 import b64encode
data = requests.post('https://api.track.toggl.com/api/v9/time_entries', json={"time_entry":{"description":"Hello Toggl","tags":["billed"],"duration":1200,"start":"1984-01-03T19:15:05.000Z","pid":123}}, headers={'content-type': 'application/json'})
print(data.json())
extern crate tokio;
extern crate serde_json;
use reqwest::{Client};
use reqwest::header::{CONTENT_TYPE};
#[tokio::main]
async fn main() -> Result<(), reqwest::Error> {
let client = Client::new();
let json = client.request(Method::POST, "https://api.track.toggl.com/api/v9/time_entries".to_string())
.json(&serde_json::json!({"time_entry":{"description":"Hello Toggl","tags":["billed"],"duration":1200,"start":"1984-01-03T19:15:05.000Z","pid":123}}))
.header(CONTENT_TYPE, "application/json")
.send()
.await?
.json()
.await?;
println!("{:#?}", json);
Ok(())
}